Website Speed Tuning
I have had to optimise this website.
Google Page Insights says that I am to slow, at least on mobile…
And since Core Web Vitalis will be ranking factor, I have decided to take action.
Before (on mobile):
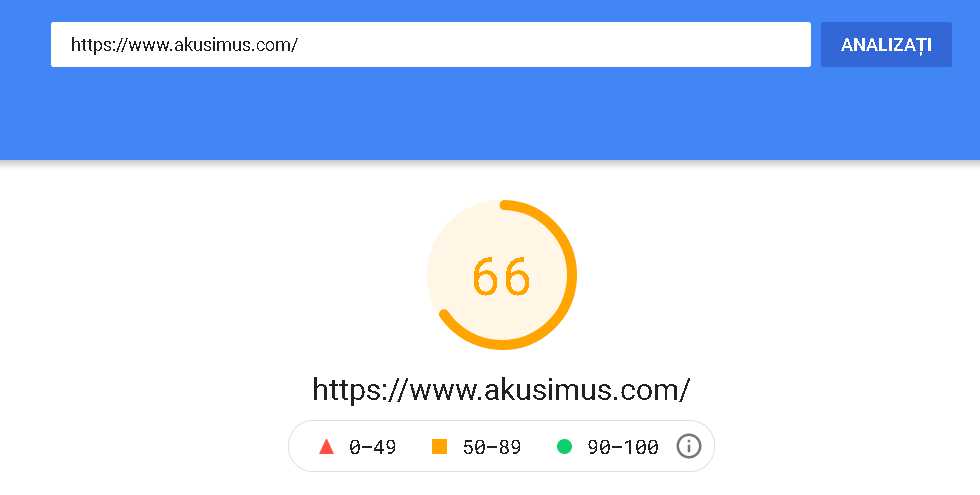
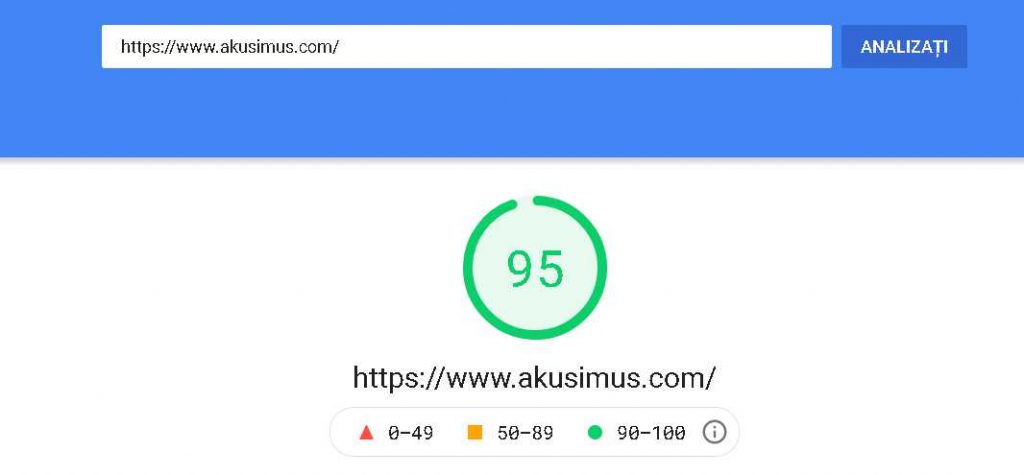
Some time later (mobile and desktop):
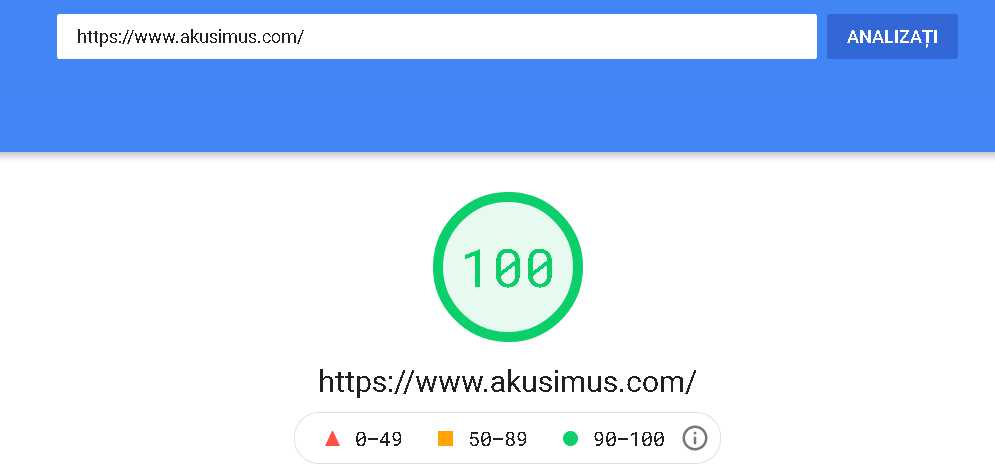
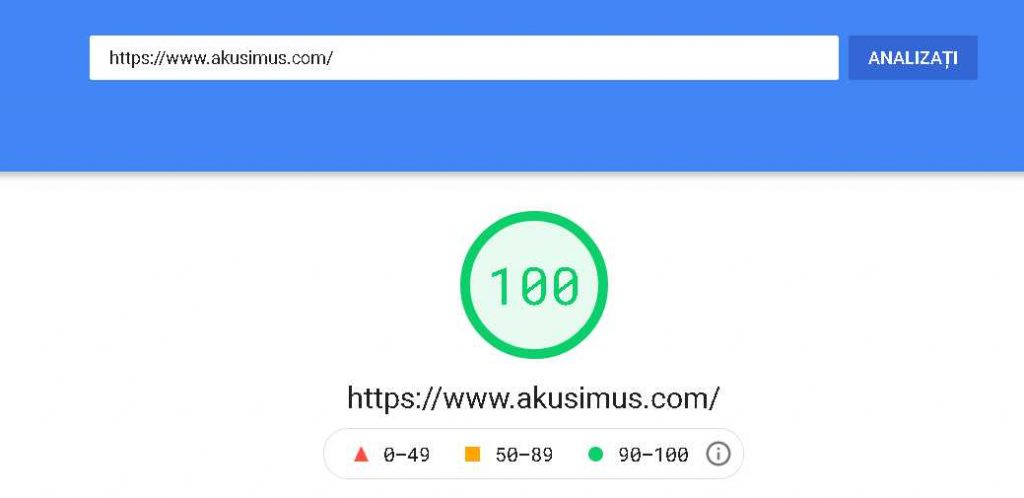
If you want the same optimisation for your website, drop me a message.
Change the MAC address of a network card
I’ve recently hit a roadblock when I had to change the MAC address of my wetwork board on a Kali Linux computer. I have written the code below in a coding language called Python. It allows you to change your MAC address easily by running a simple command in the Terminal.
#!/usr/bin/env python
import subprocess
import optparse
import re
def get_arguments():
parser = optparse.OptionParser()
parser.add_option("-i", "--interface", dest="interface", help="Interface to change its MAC address")
parser.add_option("-m", "--mac", dest="new_mac", help="New MAC address")
(options, arguments) = parser.parse_args()
if not options.interface:
parser.error("[-] Please specify an interface, use --help for more info. ")
elif not options.new_mac:
parser.error("[-] Please specify a new mac, use --help for more info. ")
return options
def change_mac(interface, new_mac):
print("[+] Changing MAC address for " + interface + " to " + new_mac)
subprocess.call(["ifconfig", interface, "down"])
subprocess.call(["ifconfig", interface, "hw", "ether", new_mac])
subprocess.call(["ifconfig", interface, "up"])
def get_current_mac(interface):
ifconfig_result = subprocess.check_output(["ifconfig", interface])
mac_adress_search_result = re.search(r"\w\w:\w\w:\w\w:\w\w:\w\w:\w\w", str(ifconfig_result))
if mac_adress_search_result:
return mac_adress_search_result.group(0)
else:
print("[-] Could not read MAC address. ")
options = get_arguments()
current_mac = get_current_mac(options.interface)
print("Current MAC = " + str(current_mac))
change_mac(options.interface, options.new_mac)
current_mac = get_current_mac(options.interface)
if current_mac == options.new_mac:
print("[+] MAC address was successfully changed to " + current_mac)
else:
print("[-] MAC address did not get changed. ")